This example shows the usage of TSxChangeNotifier
component. Use this component if you have a need to monitor file modification,
creation or deletion. The component allows you to monitor a single file
as well as a group of files, you can use masks and monitor several directories
in the folders tree. When file event occurs the TSxChangeNotifier calls
corresponding event handler and reports the name of file that was changed.
The example below shows how to use TSxChangeNotifier
to monitor all files in a single directory.
Create a new project: File -> New... ->
Application and drop the TSxChangeNotifier onto its main form. Then add
a couple of controls as shown below (you can download a ready example
using a links below):
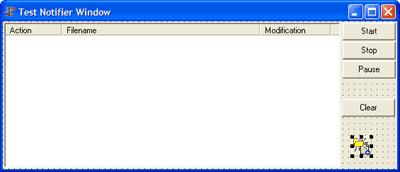
Save the project now.
The next step is a configuration of TSxChangeNotifier
properties. Use the data from the table below to set the properties:
Property Name |
Property Value and Description |
Filters |
This property contains a list of filters.
Only files that match the filter will be monitored by the component.
Current example uses the *.* mask to monitor all files in the specified
directory. |
MaxLevels |
The depth of folders tree to be monitored.
Use MaxLevels = 1 for this example. |
Root |
This property should contain a path to
the folder to be monitored. File monitoring will be performed in this
folder as well as in its subfolders. The depth of monitoring is limited
by MaxLevels and WatchSubtree properties. |
WatchSubtree |
Set this property to False to prevent
monitoring of subfolders. In other case set it to True. |
Set button's event handlers now. Code for the
"Start" button is:
procedure TTestNotifierWindow.StartClick(Sender: TObject);
begin
ChangeNotifier.Start;
end;
|
The following code should be used for the "Stop"
button:
procedure TTestNotifierWindow.StartClick(Sender: TObject);
begin
ChangeNotifier.Stop;
end;
|
The "Pause" works as:
procedure TTestNotifierWindow.btnPauseResumeClick(Sender: TObject);
begin
if not ChangeNotifier.Paused then
begin
ChangeNotifier.Paused:=True;
btnPauseResume.Caption:='Resume';
end
else
begin
ChangeNotifier.Paused:=False;
btnPauseResume.Caption:='Pause';
end;
end;
|
The next handler is for "Clear"
button:
procedure TTestNotifierWindow.StartClick(Sender: TObject);
begin
ListView.Items.Clear;
end;
|
Now it is a time to add code
to component's event handlers. All three handlers are similar. The AddFile
event should look like:
procedure TTestNotifierWindow.ChangeNotifierAddFile(Sender: TObject;
FileInfo: TSxFileInfoEventData);
var
ListItem:TListItem;
begin
ListItem:=ListView.Items.Add;
ListItem.Caption:='Created';
ListItem.SubItems.Add(FileInfo.Filename);
ListItem.SubItems.Add(DateTimeToStr(FileInfo.NewLastAccessTime));
end;
|
The TSxChangeNotifier.OnChange event is
handled as:
procedure TTestNotifierWindow.ChangeNotifierChange(Sender: TObject;
FileInfo: TSxFileInfoEventData);
var
ListItem:TListItem;
begin
ListItem:=ListView.Items.Add;
ListItem.Caption:='Modified';
ListItem.SubItems.Add(FileInfo.Filename);
ListItem.SubItems.Add(DateTimeToStr(FileInfo.OldLastWriteTime));
end;
|
And the TSxChangeNotifier.OnDelete event
should contain a code:
procedure TTestNotifierWindow.ChangeNotifierDelete(Sender: TObject;
FileInfo: TSxFileInfoEventData);
var
ListItem:TListItem;
begin
ListItem:=ListView.Items.Add;
ListItem.Caption:='Deleted';
ListItem.SubItems.Add(FileInfo.Filename);
ListItem.SubItems.Add(DateTimeToStr(FileInfo.OldLastAccessTime));
end;
|
Save the project and compile it
- it is ready now! Launch it and press the "Start" button. First
of all the application will search for files that match the mask and will
collect their data into internal database. These data will be used later
to monitor changes.
You can save this database to avoid
re-scanning when application restarts. Use the PersistentRoot è
RootImageFileName properties to do it. When the monitoring is stopped
the database is dumped into the file, when monitoring is restarted the
database will be restored. Another use of this feature - you can monitor
changes even if the monitoring process was restarted.
Use links below
to download source codes of this example as well as binary files:
Top
|