Thumbnail images that user can see
in the thumbnail view mode are produced by special type of shell extensions
known as ExtractImage Handlers. Taking this example you will learn how
to quickly implement your own thumbnail image handler.
The ExtractImage handler is an ActiveX
library like all other Shell Extensions. The first step to be done is
to create a new ActiveX Library. Create it with the following sequence
of operations: open the Repository dialog using File | New | Other...
menu, then select the ActiveX tab and click the ActiveX Library icon.
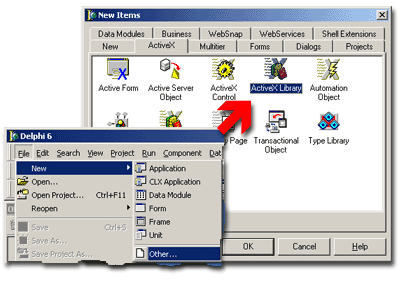
Fig. 1. New ActiveX Library creation.
Save the created project.
The next step is to add the instance of TSxDataModule
to the project. Every Shell+ component should be placed on SxDataModule
1. This special descendant of TDataModule
supplies several internal methods that allow Shell+ components to safely
operates in multiple Shell threads and automates their registration and
initialization. Note that you can place several Shell+ components onto
the same SxDataModule as well as you can add multiple TSxDataModule instances
to your project. It is easy to add new SxDataModule to the project - just
click corresponding icon in the Repository:
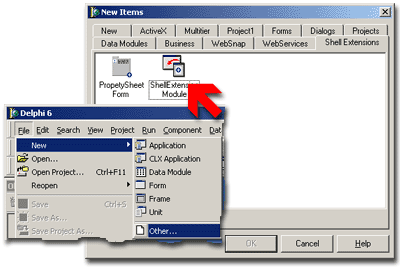
Fig. 2. How to create new SxDataModule instance.
You can save the example now.
 |
Add the TSxExtractImage
component now. This component handles shell requests of image extraction
and allows you to easy customize its behavior using a few lines
of code. As usual when you place the component onto SxDataModule
the CLSID property is set with a newly generated GUID. It is this
GUID will be used by Shell to identify your extension. Other properties
are presented in the table below:
|
Property name
|
Description
|
CLSID
|
GUID. It is used to identify the extension, t is generated
automatically when you drop component onto SxDataModule.
|
Description
|
The description of your extension, enter desired text
here.
|
ExtensionName
|
Short extension name, MyImageHandler for example.
|
FileType
|
File type to be handled by the extension. Shell will
call our extension to get thumbnail for files of the specified type
only.
|
Name
|
As usual it is a Delphi's name of component.
|
Image
|
If assigned this property is used as a static thumbnail
image. If you need to supply thumbnail dynamically then use the
OnExtractImage event. This event is considered below.
|
OverwriteExistent
|
Only one thumbnail handler can be installed for a
given file type. It is possible to override an existing thumbnail
handler when you install your own one. To avoid possible problems
you can control whether to override previous handler. If the OverwriteExistent
is true then the existing handler will be overwritten. The OnKeyAlreadyExist
event allows you to determine CLSID of the previously installed
extension to allow or prohibit overriding and/or for backup purposes.
|
Table 1. TSxExtractImage properties.
Use Object Inspector to set TSxExtractImage
properties as shown below.
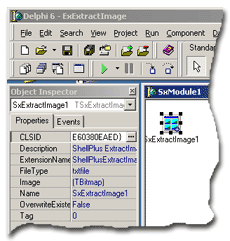
Fig 3. Setting TSxExtractImage properties
Every time shell needs the image
to display it calls a specific interface supplied by the extension library.
You have not to care about all implementation details. The only thing
you will have to do is to handle the OnExtractImage event. This
event is fired as a result of shell request. The event handler should
return the image and it is this image will be shown by the system.
Select the Events tab of the Object
Inspector and double click the OnExtractImage handler. The body of the
handler will be created by Delphi, add the following code there:
procedure TSxModule1.SxExtractImage1ExtractImage(Sender: TSxExtractImage;
Image: TBitmap; Size: TSxSize);
var
TempBitmap:TBitmap;
begin
TempBitmap:=TBitmap.Create; // create temporary image
try
// set it's size
TempBitmap.Width:=Size.cx;
TempBitmap.Height:=Size.cy;
// prepare to draw something
TempBitmap.Canvas.Font.Name:='Arial';
TempBitmap.Canvas.Font.Size:=5;
// call auxiliary drawing function, its code follows below
RenderTextFile(Sender.FileName,TempBitmap);
// return the result
Image.Assign(TempBitmap);
finally
TempBitmap.Free;
end;
end;
|
The rendering procedure is simple
enough, it draws document contents as a plain text, line by line:
procedure RenderTextFile(FileName:String;var Bitmap:TBitmap);
var
TempFile:TextFile;
i:Integer;
TempString:String;
begin
AssignFile(TempFile,FileName);
try
Reset(TempFile);
i:=0;
repeat
if not EOF(TempFile) then
ReadLn(TempFile,TempString)
else
Break;
Bitmap.Canvas.TextOut(0,i,TempString);
i:=i+Bitmap.Canvas.TextHeight('A');
until(i>Bitmap.Height);
finally
CloseFile(TempFile);
end;
end;
|
Save the project and compile it
- it is ready now! As well as any other ActiveX library the Shell+ project
requires registration. You can register it in several ways:
- from command prompt using MS regsvr32 utility: regsvr32.exe Project1.dll
- from command prompt using Borland's tregsvr utility: tregsvr Project1.dll
To know more about shell extension installation please follow
the link.
Create an empty text document in
any acceptable folder and switch the folder view to Thumbnails mode. If
the example works correctly then thumbnail image of the test document
should be empty:
Fig 4. Switch to Thumbnails view mode.
Edit the test document now and
add any text text to it. Save the changed document and in a few seconds
system will refresh the thumbnail. Now it is not clear! It contains
the text you've entered in it! The picture you see is a result of drawing
performed by RenderTextFile:
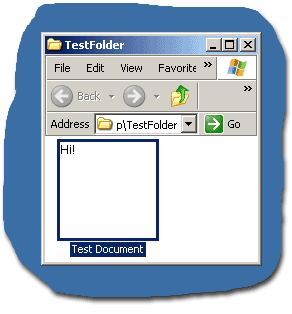
Fig. 5. Thumbnail image of document with text.
Use
links below to download source codes of this example as well as binary
files:
Top
|